Building mobile apps for both iOS and Android is no longer a luxury, but a necessity. However, developing separate native apps for each platform can be resource-intensive in terms of time, money, and effort. React Native, a framework developed by Facebook, has emerged as a powerful solution to this challenge, enabling developers to build high-performance cross-platform mobile apps using a single codebase.
This comprehensive guide will walk you through everything you need to know about building cross-platform mobile apps with React Native. From the core concepts to advanced techniques, we’ll explore why React Native is a game-changer for developers and businesses alike. Along the way, we’ll discuss best practices, look at some real-world use cases, and provide insights on optimizing React Native apps for production.
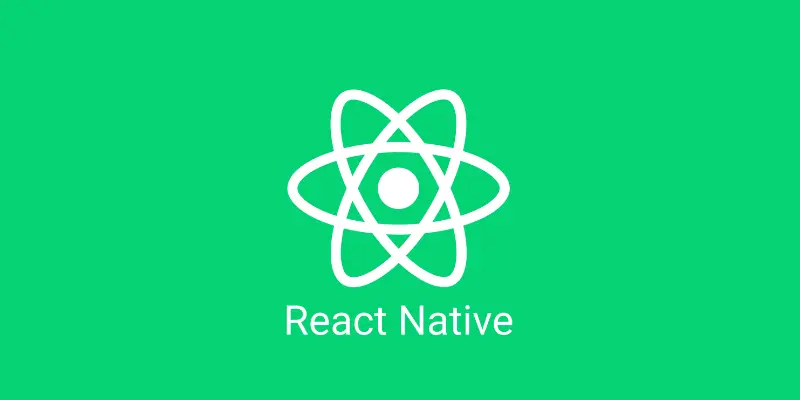
What is React Native?
React Native is an open-source framework developed by Facebook that allows developers to build mobile apps using JavaScript and React. What sets React Native apart from other mobile frameworks is that it enables developers to write code once and deploy it across both iOS and Android platforms, significantly reducing development time and costs.
Unlike other cross-platform frameworks, React Native does not rely on WebViews to render components. Instead, it compiles to native code, which allows it to deliver near-native performance and a better user experience.
Key Highlights:
- Developed by Facebook: React Native is backed by Facebook and is used in production by companies like Instagram, Airbnb, and Walmart.
- Single Codebase: Write once, run on both iOS and Android with minimal platform-specific code.
- Native Components: Offers native-like performance by rendering components using native APIs.
Why Choose React Native for Cross-Platform Development?
React Native has gained immense popularity in the mobile development community, and for good reason. It combines the efficiency of cross-platform development with the performance of native apps. Below are some compelling reasons why developers and businesses choose React Native for cross-platform app development.
Benefits of React Native:
Advantages | Description |
---|---|
Cost-Effective | With a single codebase for both iOS and Android, development and maintenance costs are reduced significantly. |
Faster Time to Market | Shared code across platforms speeds up development, enabling quicker deployment and faster iterations. |
Native-Like Performance | React Native renders components using native APIs, ensuring smooth and responsive performance compared to hybrid apps. |
Reusable Components | Components can be reused across different projects or between web and mobile applications. |
Large Ecosystem and Community | React Native has a vast library of third-party plugins and an active community, making it easier to find solutions and support. |
Hot Reloading | React Native supports hot reloading, which allows developers to see code changes in real-time without restarting the app. |
Why Businesses Love React Native:
- Efficiency: React Native allows businesses to allocate fewer resources to development while maintaining the quality of the app across platforms.
- Quicker Updates: Hot reloading and live reloading features help teams implement changes faster.
- Scalability: React Native apps are highly scalable and can handle both small and large-scale applications.
Industry Adoption
React Native is used by companies like Facebook, Instagram, Airbnb, Skype, UberEats, and more. According to a recent survey, 42% of mobile developers are using React Native, highlighting its wide adoption.
Setting Up Your React Native Environment
Getting started with React Native is straightforward, but you’ll need to set up a few tools and dependencies first. Follow these steps to set up your React Native development environment.
Prerequisites:
- Node.js: Ensure that you have the latest version of Node.js installed. React Native uses Node.js for package management.
- Watchman: Developed by Facebook, Watchman is a tool that monitors changes in the file system and is recommended for React Native.
- Xcode (for iOS development): For macOS users, you’ll need Xcode to build and run iOS apps.
- Android Studio (for Android development): Android Studio provides the necessary SDKs and tools for Android development.
Step-by-Step Installation:
- Install Node.js: Download and install Node.js from Node.js.
- Install Watchman: On macOS, you can install Watchman using Homebrew:
brew install watchman
3. Install React Native CLI:
npm install -g react-native-cli
4. Create a New React Native Project:
react-native init MyNewApp
5. Running the App on iOS:
Open the iOS folder in Xcode, and click the “Play” button to run your app on the simulator.
6. Running the App on Android:
Start an Android emulator via Android Studio, and then run the following command:
react-native run-android
Once the environment is set up, you’re ready to start building your cross-platform mobile app.
React Native vs. Native Development
One of the primary decisions businesses and developers face is whether to choose React Native or native developmentfor their mobile apps. Both approaches have their pros and cons, and the choice depends on project requirements such as performance, development speed, and budget.
Comparison Table: React Native vs. Native Development
Factor | React Native | Native Development (iOS/Android) |
---|---|---|
Code Reusability | 85%-90% code reusability across platforms | Separate codebases for iOS (Swift/Objective-C) and Android (Kotlin/Java) |
Performance | Near-native performance | High performance with direct access to native APIs |
Development Speed | Faster due to single codebase | Slower as separate development is required for each platform |
Cost | Lower development and maintenance costs | Higher costs due to multiple codebases |
Access to Native Features | Good, but may require native code for certain features | Full access to all native device features and optimizations |
Key Takeaway:
For businesses aiming to launch an app quickly on both platforms without sacrificing performance, React Native is an excellent choice. However, for apps that require maximum performance, full hardware access, or advanced native functionality, native development might still be the preferred option.
Key Features of React Native
React Native stands out among cross-platform frameworks due to several powerful features that enhance both the development experience and the app’s performance.
Hot Reloading
Hot reloading is a feature that allows developers to see changes in real-time without having to reload the entire app. This significantly speeds up development by allowing immediate feedback and faster iteration.
Native Modules
For scenarios where React Native’s JavaScript implementation doesn’t meet all the requirements, developers can integrate native modules. These modules allow you to write native code for Android (Java/Kotlin) and iOS (Objective-C/Swift) while still leveraging the benefits of React Native.
Expo: Simplifying Development
Expo is a toolchain built on top of React Native that simplifies the development process. It provides a set of APIs for camera, push notifications, geolocation, and more, without requiring native code. Expo is particularly helpful for beginners or smaller projects where time is a key factor.
Declarative Programming
React Native uses React’s declarative style of programming, which allows developers to describe how the UI should look at any given time and React Native takes care of rendering it. This results in cleaner, more predictable code, especially for complex UIs.
Best Practices for Building React Native Apps
To build robust and scalable mobile apps with React Native, developers must follow best practices that optimize the app’s performance, maintainability, and scalability.
Component-Based Architecture
Use React Native’s component-based architecture to break your UI into reusable, modular pieces. This approach not only keeps your codebase clean but also makes it easier to maintain and scale as your app grows.
State Management
For managing application state, React Native works well with libraries like Redux or Context API. For large-scale apps, Redux provides a more predictable and manageable state structure.
Optimize Images and Assets
Loading high-resolution images can slow down your app’s performance. Use image optimization tools and formats like WebP to reduce file size without compromising quality. Additionally, use the FastImage component for optimized image loading.
Use TypeScript
Adopt TypeScript in your React Native project to enforce strict typing and reduce runtime errors. TypeScript helps catch errors early in the development process, leading to more reliable code.
Handling Platform-Specific Code
While React Native allows you to share most of your codebase between iOS and Android, there will be cases where platform-specific functionality is needed. React Native provides several ways to handle platform differences effectively.
Conditional Rendering
Use Platform.OS to detect the platform and conditionally render components or apply styles. For example:
import { Platform, StyleSheet } from 'react-native';
const styles = StyleSheet.create({
button: {
...Platform.select({
ios: { backgroundColor: 'blue' },
android: { backgroundColor: 'green' },
}),
},
});
Platform-Specific Files
React Native allows you to create platform-specific files by appending .ios.js
or .android.js
to the file name. This enables you to maintain separate logic for each platform while keeping your codebase clean.
Performance Optimization in React Native
While React Native offers near-native performance, certain optimizations can improve the overall responsiveness and speed of your application.
Avoid Overuse of States
Too many stateful components can slow down your app. Keep your components stateless whenever possible and move shared state higher up the component tree.
Use Memoization
Use React’s useMemo and useCallback hooks to memoize expensive calculations and functions. This prevents unnecessary re-renders and optimizes performance.
Optimize Lists
For long lists, use FlatList or SectionList instead of ScrollView. FlatList only renders items visible on the screen, making it more efficient for handling large datasets.
Real-World Examples of React Native Apps
Several high-profile companies have chosen React Native for their mobile applications, illustrating its power and flexibility.
Popular Apps Built with React Native
Company | App | Description |
---|---|---|
Facebook Ads Manager | Facebook’s Ads Manager app is built using React Native, showcasing its power to handle complex UIs. | |
Instagram implemented React Native to streamline features between iOS and Android. | ||
Walmart | Walmart App | Walmart uses React Native to unify its mobile shopping experience across platforms. |
Bloomberg | Bloomberg News | Bloomberg adopted React Native to deliver a smooth, responsive news app on both iOS and Android. |
Uber Eats | Uber Eats | React Native powers Uber Eats’ mobile app, providing a seamless cross-platform user experience. |
These examples show that React Native is capable of handling a wide range of applications, from social media to e-commerce and real-time delivery.
Challenges and Limitations of React Native
While React Native has numerous benefits, it also comes with certain challenges and limitations. Here are a few to consider:
Performance Constraints
React Native apps may not match the performance of fully native apps, especially when it comes to memory-intensive applications such as gaming or real-time graphics processing.
Limited Access to Native APIs
Although React Native provides access to many native features, some complex platform-specific APIs may require custom native code, increasing the development effort.
Frequent Updates
React Native and its ecosystem are constantly evolving. While this brings new features and improvements, it can also lead to breaking changes or incompatibility with third-party libraries.
Future Trends in React Native Development
React Native is continuously evolving, and the future looks promising with new updates and features aimed at improving performance and developer experience.
React Native Fabric
React Native Fabric is an upcoming architecture that will improve the interaction between React Native and the native layers. It aims to make React Native more efficient and flexible by decoupling rendering from native UI updates.
Support for More Platforms
The React Native community is expanding its reach beyond mobile apps, with efforts to support platforms like macOS, Windows, and even Web. This could make React Native a true “write once, run anywhere” solution.
FAQs on building cross-platform mobile apps with React Native
What are cross-platform mobile apps with React Native?
Cross-platform mobile apps with React Native are applications that run on both iOS and Android devices using a single codebase. React Native allows developers to write code in JavaScript while leveraging native components, resulting in near-native performance across multiple platforms.
Why should I choose React Native for cross-platform mobile apps?
React Native is popular for building cross-platform mobile apps because it reduces development time and costs by using one codebase for both Android and iOS. It also offers great performance, native-like UI, and a strong developer community, making it an efficient solution for mobile app development.
How does React Native compare to native app development?
React Native allows developers to build apps that feel like native applications but with a shared codebase for both platforms. While native apps offer the highest performance and access to all device features, cross-platform mobile apps with React Native offer nearly the same performance with significant time and cost savings.
Can I access native device features in cross-platform mobile apps with React Native?
Yes, React Native provides access to native device features such as the camera, GPS, and storage through modules and APIs. If needed, developers can write custom native code to access features not available out of the box.
Are cross-platform mobile apps with React Native scalable?
Yes, React Native apps are highly scalable and can support applications of all sizes, from small prototypes to full-scale enterprise solutions. It integrates well with tools and libraries that help with app performance and scalability.
How does React Native handle updates for cross-platform mobile apps?
With cross-platform mobile apps built with React Native, updates are much easier to manage since you only need to update one codebase for both platforms. This reduces the complexity and time involved in rolling out new features or fixing bugs.
What is the performance like for cross-platform mobile apps with React Native?
While React Native may not match the absolute performance of fully native applications, it provides excellent performance for most use cases. Apps built with React Native are close to native in terms of speed, responsiveness, and UI experience.
How does React Native ensure the consistency of UI across different platforms?
React Native uses native UI components, ensuring that the app looks and feels native on both iOS and Android. With React Native, developers can also apply platform-specific customizations, ensuring a consistent but adaptable user interface.
What types of mobile applications are ideal for React Native?
Cross-platform mobile apps with React Native are ideal for a wide range of applications, including e-commerce apps, social media platforms, and real-time messaging apps. Its ability to work across platforms makes it a versatile option for most mobile development projects.
What are the best practices for building cross-platform mobile apps with React Native?
To ensure the success of cross-platform mobile apps with React Native, developers should focus on optimizing app performance, reusing components effectively, and using third-party libraries and APIs. Regular testing across devices is also crucial to maintain app quality.
Conclusion
React Native has revolutionized the way developers build cross-platform mobile applications. By providing a single codebase for both iOS and Android, React Native offers businesses a cost-effective, efficient, and scalable solution without sacrificing the user experience.
While React Native may not be the perfect fit for every use case, it’s an excellent choice for most mobile app development projects, particularly for companies looking to launch quickly across platforms. By following best practices and staying updated with the latest trends, developers can unlock the full potential of React Native to create high-performance, modern mobile applications.
Ready to build your next cross-platform mobile app with React Native? Get in touch with our team of experts to bring your vision to life with a robust, scalable cross-platform solution.